Advanced Image Processing tooklkit with Flutter
This package enhances environment variable management by adding validation, type checking, and cloud integration, making projects more secure.
NPM: https://www.npmjs.com/package/advanced-env-manager
GitHub: https://github.com/emorilebo/advanced-env-manager
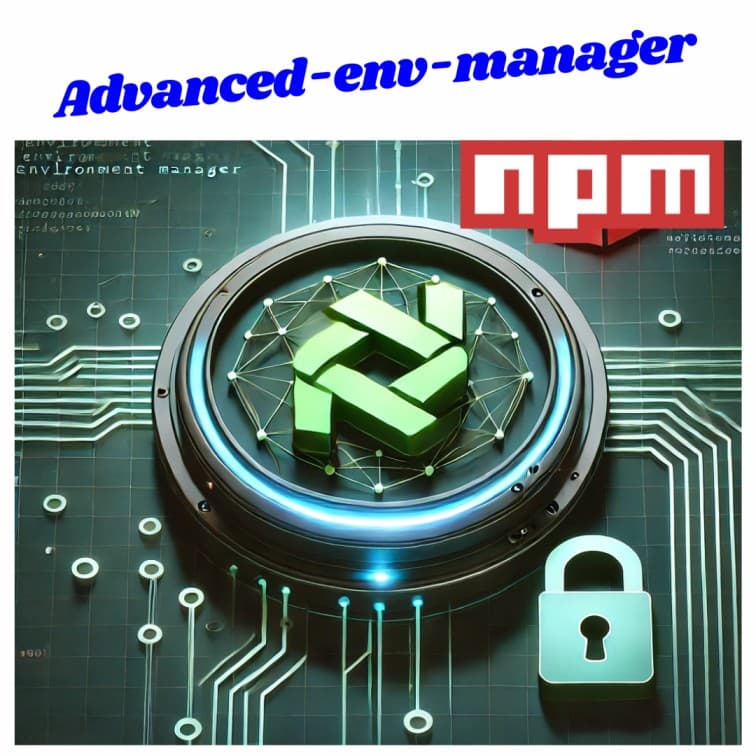
Motivation
The idea behind Advanced Environment Manager came from recurring challenges I faced while working on various Node.js projects. I noticed that many applications struggled with proper environment variable management, leading to runtime errors, security issues, and maintenance difficulties. I wanted to create a solution that would:
- Validate environment variables at startup
- Provide type safety
- Integrate seamlessly with cloud-based secret management
- Offer clear, actionable error messages
Technical Details
Core Features
-
Environment Variable Loading: The package automatically loads variables from .env files and makes them accessible via process.env. I implemented this using a combination of dotenv for file loading and custom logic for environment-specific configurations.
-
Schema Validation: I integrated Joi, a powerful validation library, to enable developers to define strict schemas for their environment variables. This ensures that all required variables are present and correctly formatted before the application starts.
-
Cloud Secret Integration: One of the most challenging aspects was implementing cloud service integration. I built a flexible system that can fetch secrets from services like AWS Secrets Manager, making it easier for teams to manage sensitive information securely.
Implementation Highlights
The core architecture of the package focuses on modularity and extensibility. Here's a simplified example of how it works:
const envSchema = Joi.object({
NODE_ENV: Joi.string().valid('development', 'production', 'test').required(),
PORT: Joi.number().default(3000),
DATABASE_URL: Joi.string().uri().required(),
}).unknown();
const env = await initEnvManager(envSchema, true);
Cloud Integration
The package supports seamless integration with AWS Secrets Manager, allowing developers to securely store and retrieve secrets from the cloud. Here's an example of how to use the AWS integration:
This simple interface masks the complexity of the underlying system, which handles:
- Multiple environment file loading
- Schema validation
- Type inference
- Cloud secret fetching
- Detailed error reporting
Challenges and Solutions
One of the biggest challenges was ensuring type safety while maintaining flexibility. I solved this by:
- Implementing a robust type inference system
- Creating detailed error messages that point directly to configuration issues
- Building a flexible plugin system for cloud service integrations
Impact and Future Development
The package has been well-received by the Node.js community, with growing adoption among developers and teams. Current development focuses on:
- Expanding cloud service integrations
- Improving performance for large configuration sets
- Adding support for runtime configuration updates
- Enhancing TypeScript integration
Lessons Learned
Building Advanced Environment Manager taught me valuable lessons about:
- Package design and API usability
- The importance of comprehensive documentation
- Balancing flexibility with safety
- Community feedback and iteration
This project represents my commitment to creating tools that make developers' lives easier while maintaining high standards for security and reliability. It's particularly satisfying to see it being used in production environments, helping teams manage their configurations more effectively.
The source code is available on GitHub, and I welcome contributions from the community. Whether you're looking to add new features, fix bugs, or improve documentation, there's always room for collaboration.